Column Moving
The Bootstrap Grid View allows you to move grid columns using drag-and-drop. To move a column, drag the column's header to the desired position.
The location in which the dragged column will be inserted after you release the left mouse button is visually indicated by two arrows.
The initial column order is specified using the column.VisibleIndex property.
By default, when you move a grid column, the grid initiates a callback to re-render the grid layout accordingly.
Setting the SettingsBehavior.ProcessColumnMoveOnClient to true allows the grid to re-render itself completely on the client, without sending a callback to the server.
First Name | Last Name | Title | Birth Date | Hire Date |
Nancy | Davolio | Sales Representative | 12/8/1978 | 5/1/2005 |
Andrew | Fuller | Vice President, Sales | 2/19/1965 | 8/14/1992 |
Janet | Leverling | Sales Representative | 8/30/1985 | 4/1/2002 |
Margaret | Peacock | Sales Representative | 9/19/1973 | 5/3/1993 |
Steven | Buchanan | Sales Manager | 3/4/1955 | 10/17/1993 |
<dx:BootstrapGridView runat="server" DataSourceID="EmployeesDataSource" KeyFieldName="EmployeeID" >
<SettingsBehavior ProcessColumnMoveOnClient="true" />
<Columns>
<dx:BootstrapGridViewDataColumn FieldName="FirstName" />
<dx:BootstrapGridViewDataColumn FieldName="LastName" />
<dx:BootstrapGridViewDataColumn FieldName="Title" />
<dx:BootstrapGridViewDataColumn FieldName="BirthDate" />
<dx:BootstrapGridViewDataColumn FieldName="HireDate" />
</Columns>
</dx:BootstrapGridView>
Column Resizing
The Bootstrap Grid View provides complete control over column availability and individual column size. End-users can easily modify column width by resizing the appropriate column header.
To resize the column, hover the right border of the column you wish to resize (so that it displays a double-sided arrow), and drag the column border.
You can access all settings related to column resizing using the SettingsResizing property.
The SettingsResizing.ColumnResizeMode property specifies how the Grid View behaves when an end-user resizes a column.
The SettingsResizing.Visualization property defines how the column resizing process is visually indicated. Two modes are available.
- In the Live mode, grid columns are redrawn dynamically during resizing.
- In the Postponed mode, grid columns are redrawn only after the operation has been completed.
The minimal column width can be set using the Settings.ColumnMinWidth or column.MinWidth property.
The Grid View can automatically truncate cell values if they don't fit into cell width. To indicate that the text is clipped, the grid displays an ellipsis ('...').
By default, this functionality is disabled, set the SettingsBehavior.AllowEllipsisInText property to true to enable it. Resize grid columns to see this feature in action.
Point the mouse cursor over an ellipsis to display a tooltip with the full text.
| | | | |
| | | | |
Maria Anders | Alfreds Futterkiste | Berlin | | Germany |
Ana Trujillo | Ana Trujillo Emparedados y helados | México D.F. | | Mexico |
Antonio Moreno | Antonio Moreno Taquería | México D.F. | | Mexico |
Thomas Hardy | Around the Horn | London | | UK |
Christina Berglund | Berglunds snabbköp | Luleå | | Sweden |
<dx:BootstrapGridView runat="server" DataSourceID="CustomersDataSource" KeyFieldName="CustomerID">
<SettingsBehavior AllowEllipsisInText="true" />
<SettingsResizing ColumnResizeMode="NextColumn" />
<Settings ColumnMinWidth="50" />
<Columns>
<dx:BootstrapGridViewDataColumn FieldName="ContactName" />
<dx:BootstrapGridViewDataColumn FieldName="CompanyName" />
<dx:BootstrapGridViewDataColumn FieldName="City" />
<dx:BootstrapGridViewDataColumn FieldName="Region" />
<dx:BootstrapGridViewDataColumn FieldName="Country" />
</Columns>
</dx:BootstrapGridView>
Header Bands
The Bootstrap Grid View ships with a multi-row header feature to help you organize grid columns into logical groups (bands).
A band is visually represented by a header displayed above the headers of the columns it combines. Each band is of a specific column type - BootstrapGridViewBandColumn. Unlike data columns, a band column is not designed to display data values directly, but to contain data (child) columns within its Columns collection. This allows you to create a hierarchy of nested bands and place a data column and a band column at the same hierarchy level.
Bands provide both display and usability benefits. They can be dragged by end-users to reorder columns. This is extremely useful if you need to provide a quick way to rearrange columns while preserving their logical grouping. Note that columns (and bands) are only allowed to move within their parent bands - you cannot move a child column from one parent band to another. This prevents end-users from breaking column grouping logic.
Drag a column header here to group by that column
Sales Person | Order |
Company | Date | Product | Qty |
Name | Country | Region | Name | Unit Price |
Steven Buchanan | Vins et alcools Chevalier | France | | 7/4/2014 | Queso Cabrales | $14.00 | 12 |
Steven Buchanan | Vins et alcools Chevalier | France | | 7/4/2014 | Singaporean Hokkien Fried Mee | $10.00 | 10 |
Steven Buchanan | Vins et alcools Chevalier | France | | 7/4/2014 | Mozzarella di Giovanni | $35.00 | 5 |
Michael Suyama | Toms Spezialitäten | Germany | | 7/5/2014 | Tofu | $19.00 | 9 |
Michael Suyama | Toms Spezialitäten | Germany | | 7/5/2014 | Manjimup Dried Apples | $42.00 | 40 |
<dx:BootstrapGridView runat="server" AutoGenerateColumns="false" KeyFieldName="OrderID"
DataSourceID="InvoicesDataSource">
<Columns>
<dx:BootstrapGridViewTextColumn FieldName="Salesperson" Caption="Sales Person" />
<dx:BootstrapGridViewBandColumn Caption="Order">
<Columns>
<dx:BootstrapGridViewBandColumn Caption="Company">
<Columns>
<dx:BootstrapGridViewTextColumn FieldName="CompanyName" Caption="Name" />
<dx:BootstrapGridViewTextColumn FieldName="Country" />
<dx:BootstrapGridViewTextColumn FieldName="Region" />
</Columns>
</dx:BootstrapGridViewBandColumn>
<dx:BootstrapGridViewDateColumn FieldName="OrderDate" Caption="Date" />
<dx:BootstrapGridViewBandColumn Caption="Product">
<Columns>
<dx:BootstrapGridViewTextColumn FieldName="ProductName" Caption="Name" />
<dx:BootstrapGridViewTextColumn FieldName="UnitPrice">
<PropertiesTextEdit DisplayFormatString="c" />
</dx:BootstrapGridViewTextColumn>
</Columns>
</dx:BootstrapGridViewBandColumn>
<dx:BootstrapGridViewTextColumn FieldName="Quantity" Caption="Qty" />
</Columns>
<CssClasses HeaderCell="text-center"></CssClasses>
</dx:BootstrapGridViewBandColumn>
</Columns>
<Settings ShowGroupPanel="true" />
<SettingsPager PageSize="5" NumericButtonCount="6"></SettingsPager>
</dx:BootstrapGridView>
Data Cell Bands
The Bootstrap Grid View provides you with a capability to organize grid columns into logical groups (bands). It allows columns to be arranged in multiple rows, and column headers and cells to occupy more than one row. A collection of a column's child columns can be accessed using the BootstrapGridViewDataColumn.Columns property.
Bands provide both display and usability benefits. Header bands can be dragged by end-users to reorder columns. This is extremely useful if you need to provide a quick way to rearrange columns while preserving their logical grouping. Note that by default, columns (and bands) are only allowed to move within their parent bands, and when a parent band is moved, it is moved along with all its children. This prevents end-users from changing the hierarchy of columns within the grids. To allow end-users to modify the column hierarchy using drag-and-drop, set the SettingsBehavior.ColumnMoveMode to ThroughHierarchy.
Photo | Address |
Features | Price |
Beds | Baths | House Size | Year Built |
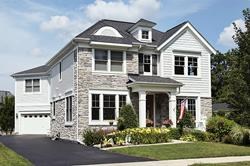 | 652 Avonwick Gate, Toronto, ON M3A25 |
Dishwasher, Disposal, Separate laundry room, 3/4 bath downstairs, Formal dining room, Downstairs family room, Separate family room, Breakfast Bar/Counter, Breakfast nook (eating area), Granite countertops in kitchen, Hardwood flooring in kitchen, Kitchen island, Solid surface countertops in kitchen, Entry foyer, Front living room, Ceiling fan in master bedroom, Master bedroom separate from other, Mirrored door closet in master bedroom, 2nd bedroom: 11x13, 3rd bedroom: 11x14, 4th Bedroom: 18x13, Alarm system owned, Built-in microwave, Carpet, Ceiling fan(s), Convection oven, Double built-in gas ovens, Gas cooktop, Gas stove, Marble/Stone floors | $780,000 |
4 | 4 | 7500 | 2008 |
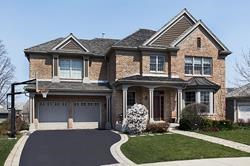 | 82649 Topeka St, Riverbank, CA 95360 |
Dishwasher, Disposal, Separate laundry room, Full bath downstairs, Formal dining room, Downstairs family room, Separate family room, Breakfast Bar/Counter, Breakfast nook (eating area), Granite countertops in kitchen, Kitchen custom cabinets, Kitchen island, Pantry, Walk-in pantry, Entry foyer, Formal living room, Rear living room, Vaulted ceiling in living room, Balcony in master bedroom, Master bedroom separate from other, Master bedroom upstairs, Sitting room in master bedroom, Walk-in closet in master bedroom, 2nd bedroom: 13X20, 3rd bedroom: 13X17, 4th Bedroom: 13X18, 5th Bedroom: 14X16, Alarm system owned, Blinds, Built-in electric oven, Built-in microwave, Carpet, Ceiling fan(s), Gas cooktop, Intercom system, Marble/Stone floors, Water conditioner owned, Water filtering system, Window Coverings Throughout | $1,750,000 |
5 | 3 | 12500 | 2009 |
<dx:BootstrapGridView runat="server" DataSourceID="HomesDataSource">
<SettingsBehavior ColumnMoveMode="ThroughHierarchy" />
<Columns>
<dx:BootstrapGridViewImageColumn FieldName="PhotoUrl" Caption="Photo">
<PropertiesImage ImageWidth="220" />
</dx:BootstrapGridViewImageColumn>
<dx:BootstrapGridViewDataColumn FieldName="Address">
<Columns>
<dx:BootstrapGridViewDataColumn FieldName="Features">
<Columns>
<dx:BootstrapGridViewDataColumn FieldName="Beds" />
<dx:BootstrapGridViewDataColumn FieldName="Baths" />
<dx:BootstrapGridViewDataColumn FieldName="HouseSize" />
<dx:BootstrapGridViewDataColumn FieldName="YearBuilt">
<CssClasses DataCell="text-end" />
</dx:BootstrapGridViewDataColumn>
</Columns>
</dx:BootstrapGridViewDataColumn>
<dx:BootstrapGridViewSpinEditColumn FieldName="Price">
<PropertiesSpinEdit DisplayFormatString="c0" />
<CssClasses DataCell="fw-bold text-center" />
</dx:BootstrapGridViewSpinEditColumn>
</Columns>
<CssClasses DataCell="fw-bold" />
</dx:BootstrapGridViewDataColumn>
</Columns>
<SettingsPager PageSize="2" NumericButtonCount="6"></SettingsPager>
<CssClasses HeaderRow="text-center" />
</dx:BootstrapGridView>